Hello Programmers, Welcome to the TheCodingHubs Bog. In this blog, we will build a QR Code Generator Using HTML, CSS & JavaScript. The technology used to construct the QR Code Generator is HTML CSS and JavaScript.
I am a programmer and content creator, having written programs and blogs since 2015, and I know how to code in HTML CSS and Javascript programming languages quickly and step by step.
Introduction of QR Code Generator Using HTML CSS & JavaScript
Many data may be stored on QR (Quick Response) codes, and users can access the information by scanning the code. Users may create a QR code for any text or URL by entering it in my QR Code Generator applications. It is not a QR code scanner but an app for creating QR codes.
We’ll utilize HTML for the web application’s structure, CSS for styling, and JavaScript for QR code creation to construct a JavaScript-based web application. We will use a package called qrcode.js to make creating QR codes easier.
You can download the source codes or files for this QR code generator at the bottom of this web page if you want to implement it.
You might like this:
- Online Ticket Booking Website Project
- Create a Chatbot with HTML CSS and JavaScript
- Make Calculator in HTML CSS and JavaScript
- Resume Generator App
Steps to Build QR Code Generator Using HTML CSS & JavaScript
The JavaScript-based QR code generator web application may be implemented in the following steps:
Step 1: Set Up Your Project Directory
Make a new folder and give your project whatever name you like. I given QR Code Generator Using HTML CSS & JavaScript. Inside this directory, create three files:
- index.html
- styles.css
- script.js
Step 2: Write the HTML Code
First, let’s set up the basic structure of our HTML page. This includes a form for the user to enter the URL, a button to submit the form, and a container to display the generated QR code. Open index.html
in your text editor and add the following code:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>QR Code Generator</title> <link rel="stylesheet" href="styles.css"> </head> <body> <div class="container"> <h1>QR Code Generator</h1> <form id="qr-form"> <input type="text" id="url-input" placeholder="Enter URL" required> <button type="submit">Generate QR Code</button> </form> <div id="qr-code"></div> </div> <script src="https://cdn.jsdelivr.net/npm/qrcode@1.4.4/build/qrcode.min.js"></script> <script src="script.js"></script> </body> </html>
In this HTML code:
- We define a form with an input field for the URL and a button to generate the QR code.
- The
div
with the IDqr-code
Is where the generated QR code will be displayed. - We include the
qrcode.min.js
library, which will help us generate the QR code and our customscript.js
File.
Step 3: Write the CSS Code
Next in QR Code Generator Using HTML CSS & JavaScript, we’ll style our QR code generator to make it look more appealing. Open styles.css
In your text editor, add the following code:
body { font-family: Arial, sans-serif; display: flex; justify-content: center; align-items: center; height: 100vh; margin: 0; background-color: #62b9ff; } .container { text-align: center; background: white; padding: 20px; border-radius: 8px; box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); width: 100%; max-width: 400px; /* Restrict max width */ box-sizing: border-box; /* Include padding in width */ } h1 { margin-bottom: 20px; } form { display: flex; flex-direction: column; align-items: center; margin-bottom: 20px; } input[type="text"] { width: 100%; padding: 10px; margin-bottom: 10px; /* Adjusted for mobile */ border: 1px solid #ccc; border-radius: 4px; } button { width: 100%; /* Full width on mobile */ padding: 10px; border: none; background-color: #007bff; color: white; border-radius: 4px; cursor: pointer; } button:hover { background-color: #0056b3; } #qr-code { margin-top: 20px; } /* Media Queries */ @media (min-width: 600px) { form { flex-direction: row; } input[type="text"] { width: 300px; margin-right: 10px; margin-bottom: 0; /* Remove margin on larger screens */ } button { width: auto; /* Auto width on larger screens */ } }
In the CSS code:
- We style the body to center the content and set a pleasant background color.
- The container is styled to have a white background, rounded corners, and a shadow for a modern look.
- The form and its elements are styled for mobile and larger screens using media queries.
Step 4: Write the JavaScript Code
Finally, we need the JavaScript code to handle the form submission and generate the QR code. Here it is:
document.getElementById('qr-form').addEventListener('submit', function(event) { event.preventDefault(); generateQRCode(); }); function generateQRCode() { const url = document.getElementById('url-input').value; if (url) { const qrCodeContainer = document.getElementById('qr-code'); qrCodeContainer.innerHTML = ''; // Clear any existing QR code // Create a new canvas element const canvas = document.createElement('canvas'); qrCodeContainer.appendChild(canvas); QRCode.toCanvas(canvas, url, { width: 200, height: 200 }, function (error) { if (error) { console.error(error); } else { console.log('QR code generated successfully'); } }); } else { alert('Please enter a URL.'); } }
In the JavaScript code:
- We add an event listener to the form to handle the submitted event. When the form is submitted, it prevents the default form submission behavior and calls the
generateQRCode
Function. - The
generateQRCode
The function retrieves the URL the user enters, clears any existing QR code, and uses the qrcode Library to generate a new QR code on a canvas element.
Step 5: Download the QR Code Library
The HTML file includes a link to the qrcode.js
library hosted on a CDN. Ensure you have an internet connection to access this library. The script tag in the HTML file looks like this:
<script src="https://cdn.jsdelivr.net/npm/qrcode@1.4.4/build/qrcode.min.js"></script>
Step 6: Run the Application
- Open your project directory. I will open QR Code Generator Using HTML CSS & JavaScript.
- Open index.html in a web browser. You should see the QR code generator interface.
- Enter a URL in the input field and click the “Generate QR Code” button.
- The generated QR code should appear below the input field.
The Output of QR Code Generator Using HTML CSS & JavaScript
Finally here is our output of the QR Code Generator Using HTML CSS & JavaScript
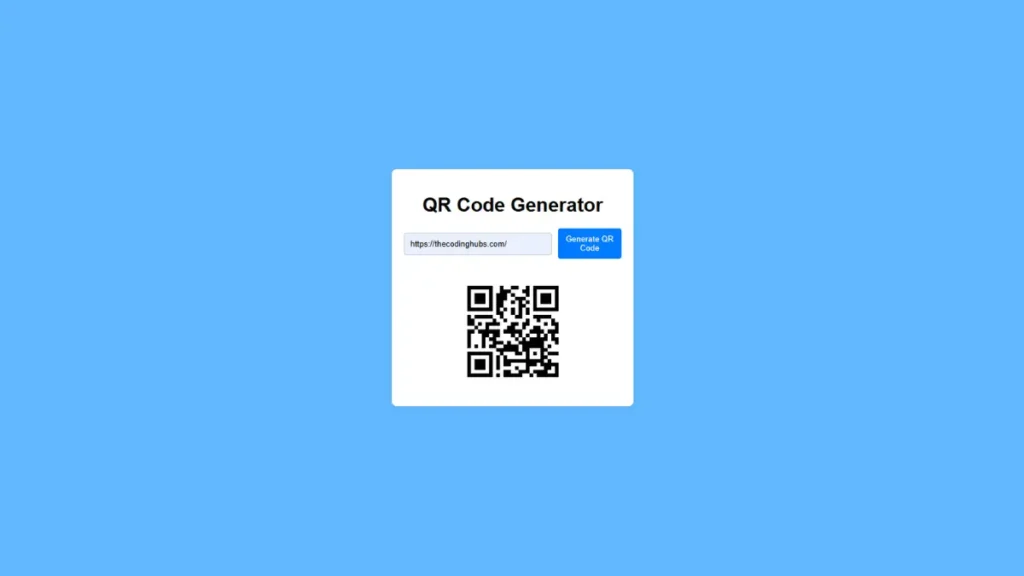
Conclusion
In this blog, I shared how to build a QR Code Generator Using HTML CSS & JavaScript. I hope you enjoyed reading this blog and found helpful information on the QR Code Generator Using HTML CSS & JavaScript. So, finally, we have completed this QR Code Generator Using HTML CSS & JavaScript. I hope this blog will benefit you. If you have any questions, related to QR Code Generator Using HTML CSS & JavaScript. you can ask me in the comment section.
nice content am a learn please can you talk more about qr generate is some how complex
Not able to create the QR code
What issue are you facing?