Introduction
In this article we are going to build YouTube Video Downloader using Python. for that we are going to use python libraries like Pytube and Tkinkter. For all those Python junkies out there, we are here with another interesting topic that equips you with the knowledge on how to craft your software for downloading videos from YouTube using Python. The reason for using Python is due to its scalability, versatility, ease of use, and adaptability. It is mostly preferred by developers for creating robust tools. Using Python’s features, we will create a YouTube video downloader in this article, enabling you to store your preferred videos locally.
Prerequisites of the Project
A few libraries are required to create the YouTube Video Downloader using Python. The following is a quick description of these libraries:
1. Pytube: The Pytube library is a lightweight, dependency-free library that helps us to download videos from YouTube.
2. Tkinter: The Tkinter library will help us to create the application with a graphical user interface (GUI).
Python already has the Tkinter library installed; it doesn’t need to be installed. So New we need to install the pytube libraries using the PIP installer by typing the following command in a command prompt or terminal.
Syntax:
# installing the pytube library $ pip install pytube
Follow the Steps To Building The YouTube video Downloader using Tkinter in Python
STEP 1: importing the modules:
from tkinter import * from pytube import YouTube
STEP 2: Initializing Tkinter:
root = Tk()
STEP 3: setting the GUI Geometry and Title:
root.geometry(“400x350") root.title(“YouTube video downloader application”)
STEP 4: defining the download function:
def download(): try: myVar.set("Downloading...") root.update() YouTube(link.get()).streams.first().download() link.set("Video downloaded successfully") except Exception as e: myVar.set("Mistake") root.update() link.set("Enter correct link")
STEP 5: Creating the label widget:
Label(root, text=”Welcome to youtubenDownloader Application”, font=”Consolas 15 bold”).pack()
STEP 6: declaring StringVar variables to store and manipulate string values:
myVar = StringVar() myVar.set(“Enter the link below”) link = StringVar()
STEP 7: Create entry widgets where the user can input the YouTube video link:
Entry(root, textvariable=myVar, width=40).pack(pady=10) Entry(root, textvariable=link, width=40).pack(pady=10)
STEP 8: Create a button entitled download that triggers the download() function when clicked:
Button(root, text=”Download video”, command=download).pack()
STEP 9: Running the Tkinter main event loop that keeps the GUI running and responsive:
root.mainloop()
Code
# importing tkinter from tkinter import * # importing YouTube module from pytube import YouTube # initializing tkinter root = Tk() # setting the geometry of the GUI root.geometry("400x350") # setting the title of the GUI root.title("Youtube video downloader application") # defining download function def download(): # using try and except to execute program without errors try: myVar.set("Downloading...") root.update() YouTube(link.get()).streams.first().download() link.set("Video downloaded successfully") except Exception as e: myVar.set("Mistake") root.update() link.set("Enter correct link") # created the Label widget to welcome user Label(root, text="Welcome to youtubenDownloader Application", font="Consolas 15 bold").pack() # declaring StringVar type variable myVar = StringVar() # setting the default text to myVar myVar.set("Enter the link below") # created the Entry widget to ask user to enter the url Entry(root, textvariable=myVar, width=40).pack(pady=10) # declaring StringVar type variable link = StringVar() # created the Entry widget to get the link Entry(root, textvariable=link, width=40).pack(pady=10) # created and called the download function to download video Button(root, text="Download video", command=download).pack() # running the mainloop root.mainloop()
Output
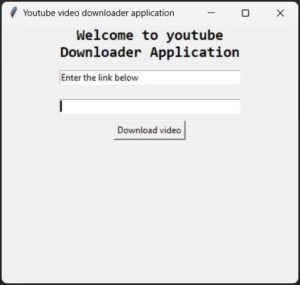
I hope that you liked reading this article and the information given to me about Python Pytube
Read More: Jai Shree Ram using Python