Table of Contents
Introduction
Hey, python junkies we are here with another super exciting topic for a real-world cause. Keeping our online stuff is very important and to do that we have to set strong passwords. After going through this article, you will be able to make your password generator using Python, a flexible programming language, and Tkinter which is a user-friendly tool that makes things look good.
Prerequisites
- Basic understanding of syntax, data types, loops, functions, and conditional statements.
- Use of modules.
- Tkinter basics for creating labels, buttons, entry fields, and combo boxes.
- Understand how to handle events such as button clicks.
MODULE used – Tkinter
Read: YouTube Video Downloader Using Python
Below is the step-by-step guide for a fully developed random password generator using Tkinter
STEP-1: Importing the modules:
import random import pyperclip from tkinter import * from tkinter.ttk import *
STEP-2: Defining the necessary functions for password generation, copying, and GUI interaction.
1. function for choosing password strength
def low(): entry.delete(0, END) # getting the length of the password length = var1.get() lower = "abcdefghijklmnopqrstuvwxyz" upper = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz" digits = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789 !@#$%^&*()" password = "" # if strength selected to be low if var.get() == 1: for i in range(0, length): password = password + random.choice(lower) return password # if strength selected to be medium elif var.get() == 0: for i in range(0, length): password = password + random.choice(upper) return password # if strength selected to be strong elif var.get() == 3: for i in range(0, length): password = password + random.choice(digits) return password else: print("Please choose an option") # function for generating the password: def generate(): password1 = low() entry.insert(10, password1) # function for copying the password to the clipboard: def copy1(): random_password = entry.get() pyperclip.copy(random_password)
STEP-3: setting up the main function for creating the GUI window:
root = Tk() var = IntVar() var1 = IntVar()
STEP-4: creating the GUI components – Title, Labels, and Entry to show password generated and for length of password:
root.title("Random Password Generator") Random_password = Label(root, text="Password") Random_password.grid(row=0) entry = Entry(root) entry.grid(row=0, column=1) c_label = Label(root, text="Length") c_label.grid(row=1)
STEP-5: creating the GUI components – Copy Button which will copy the password to the clipboard and Generate Button which will generate the password:
copy_button = Button(root, text="Copy", command=copy1) copy_button.grid(row=0, column=2) generate_button = Button(root, text="Generate", command=generate) generate_button.grid(row=0, column=3)
STEP-6: creating the GUI components – Radio Buttons for deciding the strength of the password while the default strength is set to medium:
radio_low = Radiobutton(root, text="Low", variable=var, value=1) radio_low.grid(row=1, column=2, sticky='E') radio_middle = Radiobutton(root, text="Medium", variable=var, value=0) radio_middle.grid(row=1, column=3, sticky='E') radio_strong = Radiobutton(root, text="Strong", variable=var, value=3) radio_strong.grid(row=1, column=4, sticky='E') combo = Combobox(root, textvariable=var1) #Combo box for length of your password: combo['values'] = (8, 9, 10, 11, 12, 13, 14, 15, 16, 17, 18, 19, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 30, 31, 32, "Length") combo.current(0) combo.bind('<>') combo.grid(column=1, row=1)
STEP-7: Running the GUI:
root.mainloop()
Output
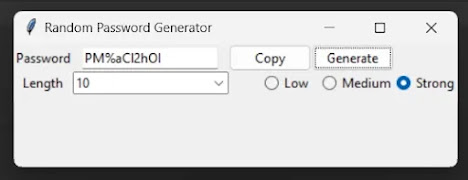
Hope this article has helped you.
Embrace yourself as, unlike others, you are trying something new 😊.
Read More Article: Jai Shree Ram using Python